[SOLVED] UART access with Ubuntu
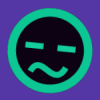
I am trying to communicate with another system via the uart on pins 8 and 10. The following doesn't seem to work even though, using a scope, I can see data rolling in on pin 10. I never see any data coming out on pin 8.
Here is my test code:
include <stdio.h>
include <stdlib.h>
include <fcntl.h>
include <unistd.h>
int main(void) {
char uptty[] = "/dev/ttyS1";
int ret = 0;
char c;
puts("!!!Hello World!!!"); /* prints !!!Hello World!!! */ int fd = open(uptty, O_RDWR | O_NOCTTY | O_NONBLOCK); if (fd < 0) { perror(uptty); exit(-1); } while (1) { // Read characters from uart. while (1) { ret = read(fd, &c, 1); if (ret == 1) write(1, &c, 1); else break; } if (ret == -1) perror("Read error"); // Write to the uart. write(fd, uptty, sizeof(uptty)); write(1, "cc\n", 3); usleep(500000); } return 0;
}
The perror() gives an "Input/output error:" if I substitute /dev/ttyS4, the error is "Resource temporarily unavailable".
Any help would be greatly appreciated. Thanks.
Comments
-
Hi @rstuart ,
First, which are you using the UP custom kernel for Ubuntu to get access to the header pins?
Then, you could try a easy test connecting RX to TX and sending an echo data to the ttyS port.
Also, you can test it using https://github.com/cbrake/linux-serial-test
Cheers!
-
Thanks for the help ccale. By consulting the https://github.com/cbrake/linux-serial-test code I was able to see that I was setting the termios structure incorrectly. (Unfortunately, the code snip-it I included in the original post did not show this part.) This was just the pointer I needed. Thanks again.
-