GPIO reading for external value
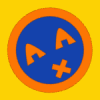
rrsuj
New Member Posts: 2 ✭
Hi,
The GPIO blink program using write operation works for me, which is described here - https://up-community.org/forum/public-ubilinux/2141-tutorial-gpio-i2c-spi-access-without-root-permissions#5724
But now I want to read signal using pin 16 (UP GPIO 18, linux GPIO 23), so I change the above mentioned program like this -
When I execute the program the value is always 1. I think after boot the gpio becomes high, so it reads value 1 always.
So I change the active_low to 1 to make the reading value to zero (I did: echo 1 > /sys/class/gpio/gpio23/active_low)
After that when I execute the program the value is always 0.
Now I connect pin1 to pin16, so that pin16 gets 3.3v, but there is no change in the readings of the program.
It still reads 0.
How can I read external signal using the GPIO in the correct way?
Please help me in this issue.
The GPIO blink program using write operation works for me, which is described here - https://up-community.org/forum/public-ubilinux/2141-tutorial-gpio-i2c-spi-access-without-root-permissions#5724
But now I want to read signal using pin 16 (UP GPIO 18, linux GPIO 23), so I change the above mentioned program like this -
#include <unistd.h> #include <stdlib.h> #include <stdio.h> #include <errno.h> #include <string.h> #include <fcntl.h> #include <getopt.h> #include <sys/ioctl.h> #include <linux/gpio.h> #include <time.h> #include <mraa.h> #define HEADERPIN 16 void sleepMillis(uint32_t millis) { struct timespec sleep; sleep.tv_sec = millis / 1000; sleep.tv_nsec = (millis % 1000) * 1000000L; while(clock_nanosleep(CLOCK_MONOTONIC, 0, &sleep, &sleep) && errno == EINTR); } int main(int argc, char **argv) { mraa_init(); mraa_gpio_context gpio; if (!(gpio = mraa_gpio_init(HEADERPIN))) { fprintf(stderr, "Error exporting pin %d!\n", HEADERPIN); mraa_deinit(); exit(1); } /* Check if the binary has root-permissions: if not, sleep for 100ms to give udev time to set the GPIO-permissions correctly for us to use the pin we just initialized above. !IMPORTANT! */ /* Try uncommenting this or changing the amount of time we sleep and see what happens. */ if(geteuid()) sleepMillis(100); if(mraa_gpio_dir(gpio, MRAA_GPIO_IN) != MRAA_SUCCESS){ fprintf(stderr, "Error setting pin-direction!\n"); mraa_gpio_close(gpio); mraa_deinit(); exit(1); } int test; while(1){ test = mraa_gpio_read(gpio); printf("value: %d\n", test); sleepMillis(1000); //Sleep one second } mraa_gpio_close(gpio); mraa_deinit(); exit(0); }
When I execute the program the value is always 1. I think after boot the gpio becomes high, so it reads value 1 always.
So I change the active_low to 1 to make the reading value to zero (I did: echo 1 > /sys/class/gpio/gpio23/active_low)
After that when I execute the program the value is always 0.
Now I connect pin1 to pin16, so that pin16 gets 3.3v, but there is no change in the readings of the program.
It still reads 0.
How can I read external signal using the GPIO in the correct way?
Please help me in this issue.
Comments
-
Hi rrsuj
The active low property doesn't change the external behaviour it only change the reported value.
So To read a 1 you have to put the pin at 0 (GND, pin 6 of the hat https://up-community.org/wiki/Pinout)
Regards
Nicola Lunghi -
Hi Nicola,
Sorry I am not getting it, I have connected pin16 to pin1 just for test.
In real environment, I have the plan to connect this input gpio to an external circuit, and the external circuit sends 0v or 1v. I need to read the 0/1 volt of the external circuit correctly through this gpio using the program running inside UP board.
So I am confused how connecting to ground can help me in real environment where the gpio will be connected to another circuit (no ground line).
How can I work in the real environment then?