MCP2515 with kernel 4.16
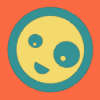
I have used a standard 4.4 kernel with UP Board HAT support patch and an RT patch Ubuntu-RT-UP-Board. I am trying to use mcp2515 for CAN Bus, I write a driver which work well.
#include <linux/init.h> #include <linux/module.h> #include <linux/spi/spi.h> #include <linux/can/platform/mcp251x.h> #include <linux/gpio.h> #include <linux/interrupt.h> int busnum = 2; int chip_select = 0; int gpio_int = 25; module_param(busnum, int, S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH); MODULE_PARM_DESC(busnum, "busnum of spi bus to use"); module_param(gpio_int, int, S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH); MODULE_PARM_DESC(gpio_int, "linux gpio number of INT gpio"); module_param(chip_select, int, S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH); MODULE_PARM_DESC(chip_select, "spi chip select"); int gpio_requested = 0; struct spi_device * dev1; static struct mcp251x_platform_data mcp251x_info = { .oscillator_frequency = 16000000, }; static struct spi_board_info spi_device_info = { .modalias = "mcp2515", .platform_data = & mcp251x_info, .irq = -1, .max_speed_hz = 10 * 1000 * 1000, }; static int __init mcp2515_init(void) { int ret; struct spi_master * master; printk("mcp2515: init\n"); ret = gpio_request(gpio_int, "sysfs"); if (ret) { printk("mcp2515: could not request gpio %d\n", gpio_int); gpio_free(gpio_int); return ret; } gpio_requested = 1; gpio_direction_input(gpio_int); ret = gpio_to_irq(gpio_int); printk("mcp2515: irq for pin %d is %d\n", gpio_int, ret); spi_device_info.irq = ret; spi_device_info.bus_num = busnum; spi_device_info.chip_select = chip_select; master = spi_busnum_to_master(spi_device_info.bus_num); if (!master) { printk("mcp2515: MASTER not found.\n"); ret = -ENODEV; goto error_postgpio; } // create a new slave device, given the master and device info dev1 = spi_new_device(master, & spi_device_info); if (!dev1) { printk("mcp2515: FAILED to create slave.\n"); ret = -ENODEV; goto error_postgpio; } printk("mcp2515: device created!\n"); return 0; error_postgpio: gpio_free(gpio_int); return ret; } static void __exit mcp2515_exit(void) { printk("mcp2515: exit\n"); if (dev1) { spi_unregister_device(dev1); } if (gpio_requested) gpio_free(gpio_int); } module_init(mcp2515_init); module_exit(mcp2515_exit); MODULE_LICENSE("GPL"); MODULE_AUTHOR("One"); MODULE_DESCRIPTION("MCP2515");
But I want to use UP Squared, and I try the kernel 4.15/4.16 with the driver I write on UP Board, but when I load the MCP2515 withsudo modprobe mcp2515
and sudo ip link set can0 type can bitrate 1000000
everything since ok. But when I type sudo ip link set up can0
and enter, the whole system crash. I got (sorry for the camera view, the system is crash):
So anybody has a similar work and suggestion?
Comments
-
Hi @QiayuanLiao
If you are using UP Squared and you are interfacing the device via SPI, then you need to check the following: https://wiki.up-community.org/Pinout_UP2#SPI_Ports
SPI ports number is changing and you might need to add the ACPI override in order to use your driver and maybe other changes too.
Also there is an official driver for that in the linux kernel, have you tried if that works?
https://github.com/torvalds/linux/blob/master/drivers/net/can/spi/mcp251x.c
-
@DCleri said:
Also there is an official driver for that in the linux kernel, have you tried if that works?https://github.com/torvalds/linux/blob/master/drivers/net/can/spi/mcp251x.c
@QiayuanLiao is actually using this driver, see: https://forum.up-community.org/discussion/96/canbus-hat-support
But because this driver uses Device Tree to get parameters and there is no progress on ACPI SSDT as mentioned here , we still have to write a (small) kernel module to use it.
-
Yes, I write a small kernel module that I have posted above. It works on kernel 4.4 with UP Board (Not UP Squared!), when I test on kernel 4.16, the issue above happened.
-
That solution was reported to work for UP Squared and latest kernel.
@QiayuanLiao have you tried what has been provided on that topic?
@BenSpex said:
So if anyone else hast the problem I have found the solution:
From @WereCatf
https://forum.up-community.org/discussion/2415/cant-get-fbtft-device-working-ubilinux -
I am trying kernel 4.9 now. It works on both UP Board and UP Squared after I patch the meta-up-board pyro, and LEDs, SPI, GPIO, PWM all work. But when I use full Fully Preemptible Kernel (RT), the system will freeze easily.
-
The problem seems became "real-time 4.9 kernel freeze on UP Board". I have met the same problem when using ubilinux (now ubuntu 16.04 or 18.04)
-
It's good news that it works with a normal kernel (not realtime).
Have you tried with Sumo on Kernel 4.14?
Are you following the suggestions from WereCatf?
-
@DCleri said:
It's good news that it works with a normal kernel (not realtime).Have you tried with Sumo on Kernel 4.14?
Are you following the suggestions from WereCatf?
I didn't follow the suggestions from WereCatf. I mean I am working on UP Board, not UP Squared now.
My workflow is as follow:
1. kernel 4.4 with RT, HAT, and MCP2515. All work on UP Board, but HAT unable to work on UP Squared.
2. kernel 4.15 with RT, HAT, and MCP2515. HAT work on UP Board, but when using MCP2515, the whole system freeze. So I didn't try on UP Squared
3. kernel 4.9 with RT, HAT, and MCP2515. RT patch leads to system freeze on UP Board. So I didn't try on UP Squared